Webhooks
Lemon Squeezy’s webhooks notify your application of key store events, such as license key creation or subscription expiration, enabling efficient handling of asynchronous activities.
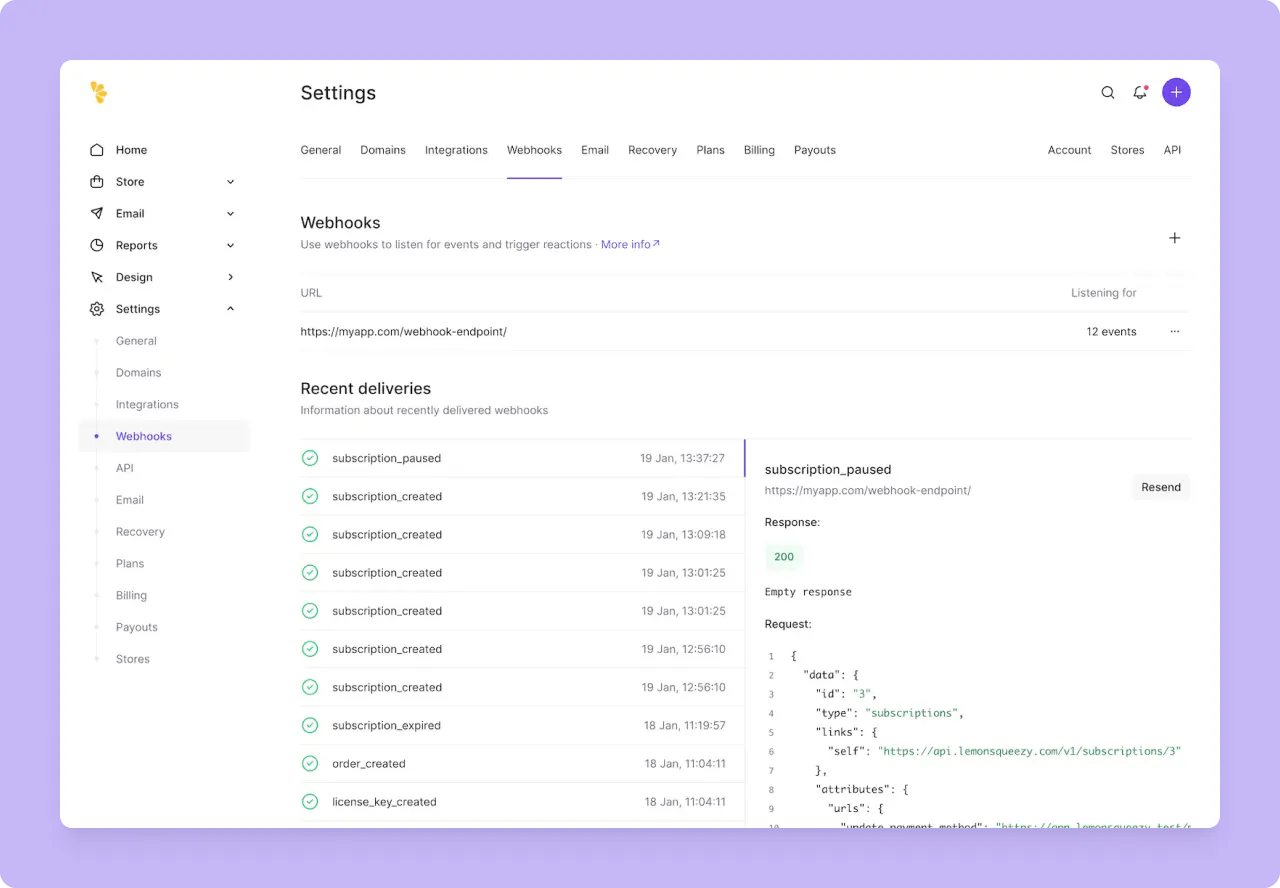
Creating webhooks
Webhooks can be set up and managed from Settings » Webhooks in your dashboard or programatically using the Lemon Squeezy API.
Webhooks require three elements to function:
Callback URL
This is the URL that Lemon Squeezy will send a POST
request to when an event is triggered.
Signing secret
This is a secret (usually a random string) between 6 and 40 characters that will be used to sign each request. You should validate against this secret on each incoming webhook so you can verify that the request came from Lemon Squeezy.
List of events
This is a list of events that will trigger this webhook.
Sending custom data
You can pass custom data through the checkout (for example, user identifiers) that can be captured using webhooks.
Read the Taking payments section of our Developer guide for a detailed example.
Viewing webhook events
Recent webhooks sent from your store are logged on the webhooks settings page. You can see which events have been sent, the full payloads of each request and also resend webhooks if you need to.